1.创建子模块
这里我们创建一个子模块
1 2
| group = 'com.ray.study' artifact ='spring-boot-03-freemarker'
|
2.引入依赖
2.1 继承父工程依赖
在父工程spring-boot-seeds
的 settings.gradle
加入子工程
1 2 3 4 5
| rootProject.name = 'spring-boot-seeds' include 'spring-boot-01-helloworld' include 'spring-boot-02-restful-test' include 'spring-boot-03-thymeleaf' include 'spring-boot-03-freemarker'
|
这样,子工程spring-boot-03-freemarker
就会自动继承父工程中subprojects
`函数里声明的依赖,主要包含如下依赖:
1 2 3 4 5
| implementation 'org.springframework.boot:spring-boot-starter-web' testImplementation 'org.springframework.boot:spring-boot-starter-test'
compileOnly 'org.projectlombok:lombok' annotationProcessor 'org.projectlombok:lombok'
|
2.2 引入freemarker
依赖
直接依赖 spring-boot-starter-freemarker
即可
将子模块spring-boot-03-freemarker
的build.gradle
修改为如下内容:
1 2 3 4
| dependencies { implementation 'org.springframework.boot:spring-boot-starter-freemarker' }
|
3.修改配置
3.1 freemarker
默认配置
FreeMarkerProperties
类及其父类中设置了 freemarker
默认配置,如下所示:
1 2 3 4 5 6 7 8 9 10
| @ConfigurationProperties(prefix = "spring.freemarker") public class FreeMarkerProperties extends AbstractTemplateViewResolverProperties {
public static final String DEFAULT_TEMPLATE_LOADER_PATH = "classpath:/templates/";
public static final String DEFAULT_PREFIX = "";
public static final String DEFAULT_SUFFIX = ".ftl"; }
|
由ThymeleafProperties
类及其父类,默认配置了
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| spring: freemarker: cache: false charset: UTF-8 enabled: true content-type: text/html template-loader-path: classpath:/templates/ prefix: 空串 suffix: .ftl
|
关于默认配置也可在spring-boot-autoconfigure
工程下的的spring-configuration-metadata.json
文件中查看
3.2 修改application.yml
1 2 3 4 5 6
| server: port: 8088 servlet: context-path: /
|
4.业务实现
所谓模板引擎,就是模板+数据
这里我们将实现一个显示用户列表的功能
4.1 准备数据
4.1.1 model
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.ray.study.springboot03freemarker.model;
import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor;
@Data @NoArgsConstructor @AllArgsConstructor public class User {
private Long id;
private String name;
private Integer age; }
|
4.1.2 controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| package com.ray.study.springboot03freemarker.controller;
import com.ray.study.springboot03freemarker.model.User; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.servlet.ModelAndView;
import java.util.ArrayList; import java.util.List;
@Controller @RequestMapping(value = "/users") public class UserController {
@GetMapping("/index1") public ModelAndView index1() {
List<User> userList = listUser();
ModelAndView view = new ModelAndView(); view.setViewName("index");
view.addObject("title", "SpringBoot 整合 Freemarker"); view.addObject("userList", userList);
return view; }
@GetMapping("/index2") public String index2(Model model) { List<User> userList = listUser();
model.addAttribute("title", "SpringBoot 整合 Freemarker"); model.addAttribute("userList", userList);
return "index"; }
private List<User> listUser(){ List<User> userList = new ArrayList<>(); for (int i=0 ; i<3; i++){ userList.add(new User(1L+i,"tom"+i,21+i)); }
return userList; }
}
|
4.2 准备模板
在src/main/resources
下创建templates
目录,并在此目录下创建index.ftl
,内容如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <!DOCTYPE html>
<html lang="en"> <head> <meta charset="UTF-8"> <title> ${title} </title> </head> <body> <h1>${title}</h1>
<table> <tr> <th>id</th> <th>name</th> <th>age</th> </tr> <#list userList as user> <tr> <td>${user.id}</td> <td>${user.name}</td> <td>${user.age}</td> </tr> </#list> </table>
</body> </html>
|
5.最终效果
启动项目,然后访问如下两个网址
会出现下图页面:
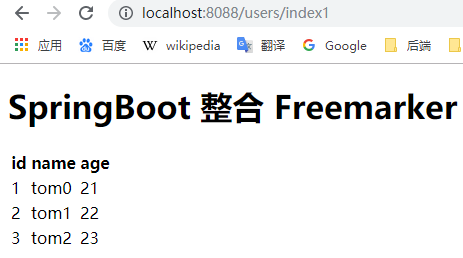